Understanding Redux - For Absolute Redux Beginners
The official documentation for Redux reads: Redux is a predictable state container for JavaScript apps.
Explaining Redux: For Absolute Redux Beginners
These bank terms are used in the article that is equivalent to Redux terms:
1. Bank Vault = Redux Store
2. Intent(i.e. withdraw money) = Redux action
3. Cashier = Redux Reducer
4. Money = State
Let’s consider an event you’re likely familiar with — going to the bank to withdraw cash. Even if you don’t do this often, you’re likely aware of what the process looks like.
You wake up one morning, and head to the bank as quickly as possible. While going to the bank there’s just one intention / action you’ve got in mind: to
When you get into the bank, you then go straight to the Cashier to make your request known.
Wait, you went to the Cashier? Why didn’t you just go into the bank vault to get your money?
Well, like you already know, things don’t work that way. Yes, the bank has money in the vault, but you have to talk to the Cashier to help you follow a due process for withdrawing your own money.
The Cashier, from their computer, then enters some commands and delivers your cash to you. Easy-peasy.
Now, how does Redux fit into this story?
We’ll get to more details soon, but first, the terminology.
1. The Bank Vault is to the bank what the
The bank vault keeps the money in the bank, right?
Well, within your application, you don’t spend money. Instead, the
Just like the bank vault keeps your money safe in the bank, the
The Redux Store can be likened to the Bank Vault. It holds the state of your application — and keeps it safe.
This leads to the first Redux principle:
2. Go to the bank with an
If you’re going to get any money from the bank, you’re going to have to go in with some intent or action to withdraw money.
If you just walk into the bank and roam about, no one’s going to just give you money. You may even end up been thrown out by the security. Sad stuff.
The same may be said for Redux.
Write as much code as you want, but if you want to update the state of your Redux application (like you do with
Now, this leads to Redux principle #2.
When you walk to the bank, you go there with a clear action in mind. In this example, you want to withdraw some money.
If we chose to represent that process in a simple Redux application, your action to the bank may be represented by an object.
One that looks like this:
Whenever you need to change/update the state of your Redux application, you need to dispatch an action.
Don’t stress over how to do this yet. I’m only laying the foundations here. We’ll delve into lots of examples soon.
3. The Cashier is to the bank what the
Alright, take a step back.
Remember that in the story above, you couldn’t just go straight into the bank vault to retrieve your money from the bank. No. You had to see the Cashier first.
Well, you had an action in mind, but you had to convey that action to someone — the Cashier — who in turn communicated (in whatever way they did) with the vault that holds all of the bank’s money.
The same may be said for Redux.
Like you made your action known to the Cashier, you have to do the same in your Redux application. If you want to update the state of your application, you convey your
This process is mostly called dispatching an
The
In Redux terms, the money you spend is your
And this leads to the last Redux principle:
With this analogy, you should now have an idea of what the most important Redux actors are: the
These three actors are pivotal to any Redux application. Once you understand how they work, the bulk of the deed is done.
Summary:
When working with Redux, you will need three main things:
1. actions: these are objects that should have two properties, one describing the type of action, and one describing what should be changed in the app state.
2. reducers: these are functions that implement the behavior of the actions. They change the state of the app, based on the action description and the state change description.
3. store: it brings the actions and reducers together, holding and changing the state for the whole app — there is only one store.
Redux Principles
1) ONE application STATE OBJECT managed by ONE STORE. Have a single source of truth: The state of your whole application is stored in an object tree within a single Redux store.
2) State is read-only: The only way to change the state is to emit an action, an object describing what happened.
3) To specify how the state tree is transformed by actions, you write pure reducers.
Source: Understanding Redux: The World’s Easiest Guide to Beginning Redux by Ohans Emmanuel
Explaining Redux: For Absolute Redux Beginners
These bank terms are used in the article that is equivalent to Redux terms:
1. Bank Vault = Redux Store
2. Intent(i.e. withdraw money) = Redux action
3. Cashier = Redux Reducer
4. Money = State
Let’s consider an event you’re likely familiar with — going to the bank to withdraw cash. Even if you don’t do this often, you’re likely aware of what the process looks like.
You wake up one morning, and head to the bank as quickly as possible. While going to the bank there’s just one intention / action you’ve got in mind: to
WITHDRAW_MONEY
.When you get into the bank, you then go straight to the Cashier to make your request known.
Wait, you went to the Cashier? Why didn’t you just go into the bank vault to get your money?
Well, like you already know, things don’t work that way. Yes, the bank has money in the vault, but you have to talk to the Cashier to help you follow a due process for withdrawing your own money.
The Cashier, from their computer, then enters some commands and delivers your cash to you. Easy-peasy.
Now, how does Redux fit into this story?
We’ll get to more details soon, but first, the terminology.
1. The Bank Vault is to the bank what the
Redux Store
is to Redux.The bank vault keeps the money in the bank, right?
Well, within your application, you don’t spend money. Instead, the
state
of your application is like the money you spend. The entire user interface of your application is a function of your state.Just like the bank vault keeps your money safe in the bank, the
state
of your application is kept safe by something called a store
. So, the store keeps your 'money' or state
intact.The Redux Store can be likened to the Bank Vault. It holds the state of your application — and keeps it safe.
This leads to the first Redux principle:
Have a single source of truth: The state of your whole application is stored in an object tree within a single Redux store.In simple terms, with Redux, it is is advisable to store your application state in a single object managed by the Redux
store
. It’s like having one vault
as opposed to littering money everywhere along the bank hall.
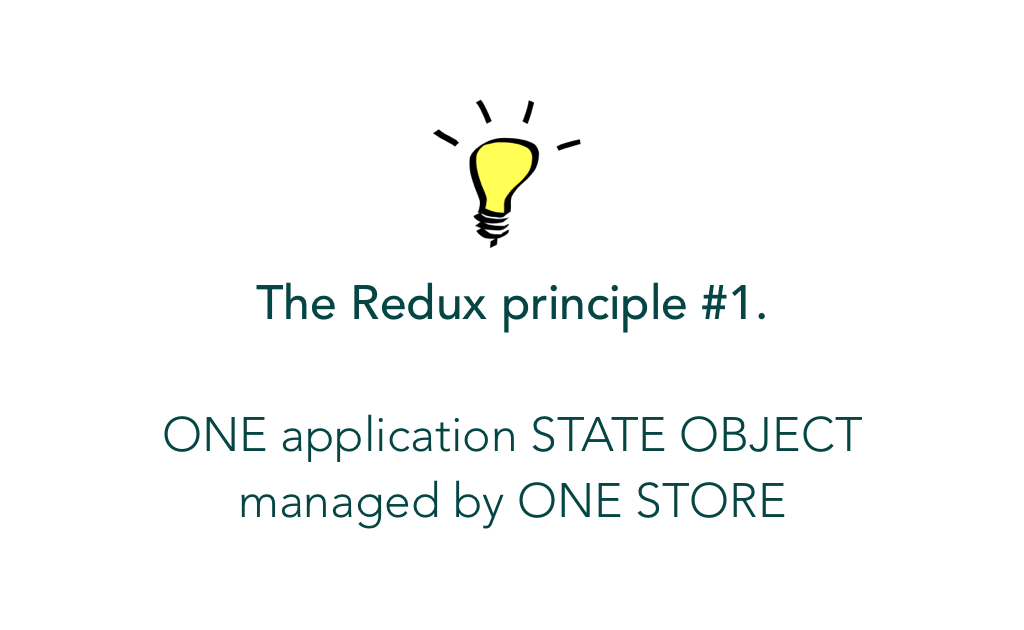
action
in mind.If you’re going to get any money from the bank, you’re going to have to go in with some intent or action to withdraw money.
If you just walk into the bank and roam about, no one’s going to just give you money. You may even end up been thrown out by the security. Sad stuff.
The same may be said for Redux.
Write as much code as you want, but if you want to update the state of your Redux application (like you do with
setState
in React), you need to let Redux know about that with an action
.Now, this leads to Redux principle #2.
State is read-only: The only way to change the state is to emit an action, an object describing what happened.What does that mean in plain language?
When you walk to the bank, you go there with a clear action in mind. In this example, you want to withdraw some money.
If we chose to represent that process in a simple Redux application, your action to the bank may be represented by an object.
One that looks like this:
{ type: "WITHDRAW_MONEY", amount: "$10,000" }In the context of a Redux application, this object is called an
action
. It always has a type field
that describes the action you want to perform. In this case, it is WITHDRAW_MONEY
.Whenever you need to change/update the state of your Redux application, you need to dispatch an action.
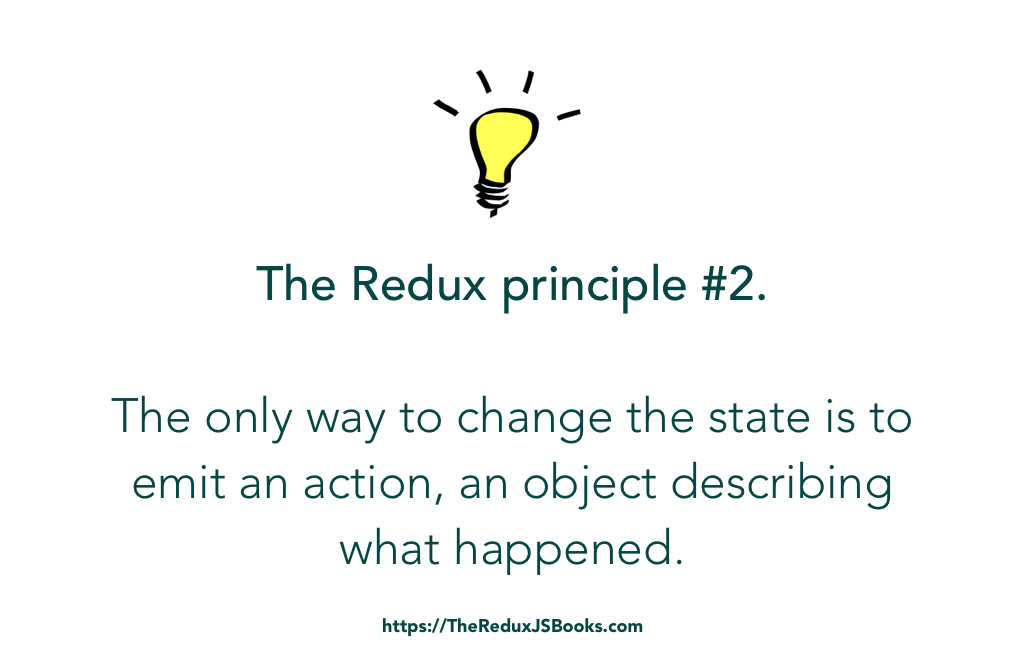
3. The Cashier is to the bank what the
reducer
is to Redux.Alright, take a step back.
Remember that in the story above, you couldn’t just go straight into the bank vault to retrieve your money from the bank. No. You had to see the Cashier first.
Well, you had an action in mind, but you had to convey that action to someone — the Cashier — who in turn communicated (in whatever way they did) with the vault that holds all of the bank’s money.
The same may be said for Redux.
Like you made your action known to the Cashier, you have to do the same in your Redux application. If you want to update the state of your application, you convey your
action
to the reducer
— our own Cashier.This process is mostly called dispatching an
action
. In this example, and in the Redux world, it is used to mean sending off the action to the reducers.The
reducer
knows what to do. In this example, it will take your action to WITHDRAW_MONEY
and ensure that you get your money.In Redux terms, the money you spend is your
state
. So, your reducer
knows what to do, and it always returns your new state
.And this leads to the last Redux principle:
To specify how the state tree is transformed by actions, you write pure reducers.As we proceed, I’ll explain what a “pure” reducer means. For now, what’s important is to understand that, to update the state of your application (like you do with
setState
in React,) your actions must always be sent off (dispatched) to the reducers to get your new state
.
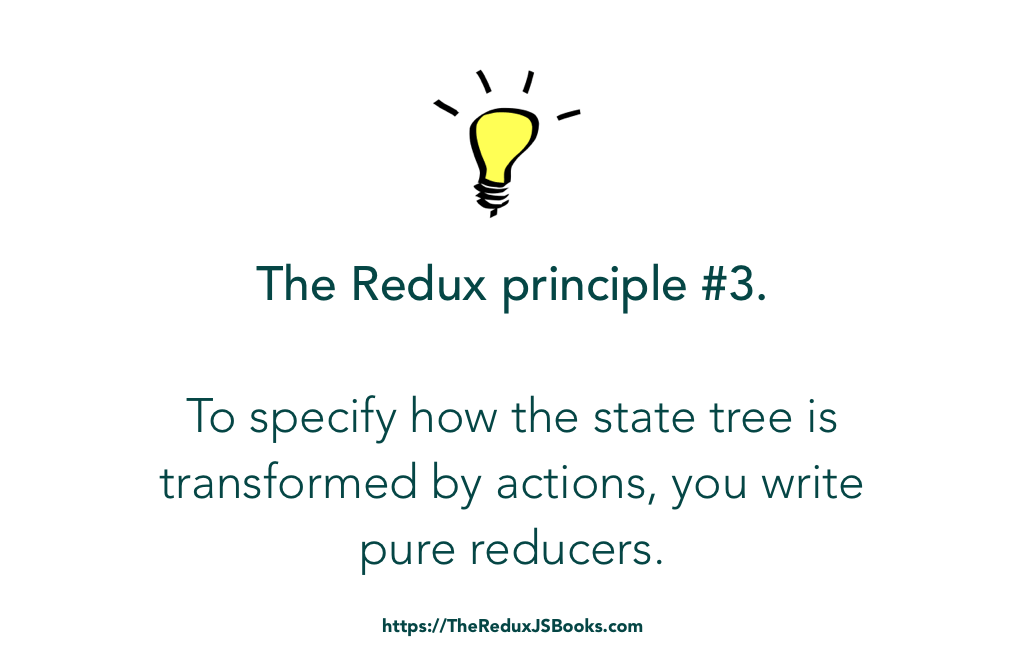
store
, the reducer
and an action
.These three actors are pivotal to any Redux application. Once you understand how they work, the bulk of the deed is done.
Summary:
When working with Redux, you will need three main things:
1. actions: these are objects that should have two properties, one describing the type of action, and one describing what should be changed in the app state.
2. reducers: these are functions that implement the behavior of the actions. They change the state of the app, based on the action description and the state change description.
3. store: it brings the actions and reducers together, holding and changing the state for the whole app — there is only one store.
Redux Principles
1) ONE application STATE OBJECT managed by ONE STORE. Have a single source of truth: The state of your whole application is stored in an object tree within a single Redux store.
2) State is read-only: The only way to change the state is to emit an action, an object describing what happened.
3) To specify how the state tree is transformed by actions, you write pure reducers.
Source: Understanding Redux: The World’s Easiest Guide to Beginning Redux by Ohans Emmanuel
Comments
Post a Comment